Developers can update specific JSON data elements fast and effortlessly thanks to the robust JSONPath tester. It is helpful for working with data and creating apps nowadays because it is simple to learn and functions well with complex JSON forms. JSONPath can be used to locate specific data in JSON documents. It makes it possible to collect data properly and directly, which is crucial for increasing the output and pace of software development.
JSONPath Definition and Overview
The JSONPath query language is designed to get data from JSON (JavaScript Object Notation), a simple data exchange format. JSONPath makes changing and accessing specific JSON data segments without having to traverse the entire data structure simple and powerful automatically. It was based on the XML syntax known as XPath.
In JSONPath syntax, expressions trailing the JSON structure come after the root object or array. It enables filters, array slice operators, and wildcards to find the necessary data. It is beneficial when working with complicated or sizable JSON objects.
JSONPath’s Significance in Data Processing and Manipulation
For several reasons, JSONPath is essential to contemporary data handling and manipulation.
- Effectiveness: Using JSONPath expressions to extract specific data from a JSON structure is significantly more efficient than natively parsing a JSON object or array. Performance can be increased considerably, and the required code can be reduced, especially for extensive JSON data.
- Adaptability: JSONPath can be used to modify data in both front-end and back-end applications, and it can be utilized in various computer environments and technologies. JavaScript, Python, PHP, and most other programming languages that support JSON can all use it.
- Testing and Debugging: To ensure that APIs return the proper data structure and content, JSONPath can validate JSON outputs in software testing. Due to this, it’s a crucial tool for creating dependable and durable applications.
JSONPath Testers Overview
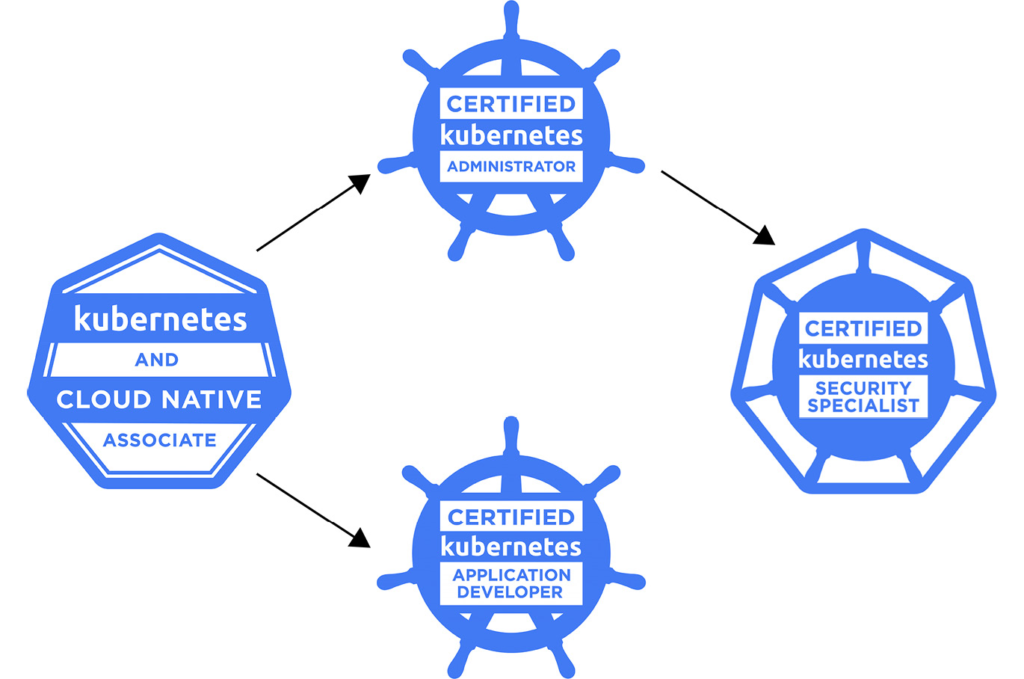
To ensure that your JSONPath searches function with actual JSON data, you can utilize software or web tools known as JSONPath simulators. Developers require these testers to promptly verify that their JSONPath expressions are correctly written and produce the desired data.
Essential Features of JSONPath Testers
- Interactive Interface: Most JSONPath testers provide an easy-to-use interface to create JSONPath queries and enter JSON data. Users can refine their inquiries iteratively with the instantaneous presentation of query results.
- Real-time Data: Several testers offer real-time results as you create or edit your JSONPath expression, showing which data is chosen from the JSON input. JSONPath query optimization and debugging benefit considerably from this rapid input.
- Highlighting Syntax: JSONPath users frequently apply syntax highlighting to JSON data and JSONPath searches to make things easier to read and use. It facilitates visually distinguishing between elements, characteristics, and values.
- Error Handling Capacity: When a JSONPath query contains a grammatical error, good tests for JSONPath should display error messages or more information. This aids in problem-solving and helps you learn the correct code.
- Execution of Numerous Queries: A few sophisticated testers permit the simultaneous execution of many JSONPath queries against the same JSON data. This capability is handy when comparing outcomes or handling intricate data extraction jobs.
- Easy Reporting: A few JSONPath testers allow users to share their JSON and JSONPath configurations using a URL, facilitating easy collaboration and question-specific assistance.
Typical Applications for JSONPath Testers
The following are some typical applications for JSONPath testers:
- Debugging JSONPath queries: Developers utilize JSONPath testers to improve and solve flaws in their searches to ensure they return accurate results.
- Understand and Experiment: Testers allow new users to become familiar with JSONPath by enabling them to understand the code and observe how different questions affect the output.
- API Testing and Validation: Before adding API responses to their programs, developers can employ JSONPath testers to ensure the data extraction logic is sound.
- Data Examination: Testers can also be used to investigate patterns in JSON that consumers are unaware of. This aids in their understanding of the data’s structure and arrangement.
Configuring Your Environment for Testing JSONPath
To ensure you can develop, test, and debug JSONPath queries quickly and effectively, you must set up your environment for JSONPath testing. It entails choosing the appropriate tools and configuring them. The following instructions will walk you through setting up a productive environment for JSONPath testing:
1. Select a Tester for JSONPath
The first step is to choose a JSONPath tester that works for you. As was previously noted, there are a variety of online testers accessible, such as the JSONPath Online Evaluator and JsonPath.com. Tools like Postman and Advanced REST Client offer extensive API and JSONPath testing capabilities for more integrated solutions.
2. Set Up the Tools for Development
It may be necessary to install specific programs or libraries if you would like to test JSONPath searches locally or as part of a development project:
- Use an Integrated Development Environment (IDE): Use a Text editor or IDE that supports JSON and JavaScript syntax, such as WebStorm or Eclipse, or a text editor like VSCode or Sublime. These technologies frequently provide built-in capability for handling JSON through plugins.
- Using JSONPath Libraries: Install a library that supports JSONPath based on your programming language. For example:
- JavaScript: Use npm to install libraries such as jsonpath.
- Python: Make use of jsonpath-ng or jsonpath-rw, which pip may install.
- Java: You can use Maven or Gradle to incorporate libraries like Jayway JsonPath in your project.
3. Set Up Your Code Editor or IDE
Set up your text editor or IDE to handle JSON and JSONPath as efficiently as possible:
- Plugins and extensions: Include plugins that simplify the authoring of JSON, such as those that check for problems, display grammar, and automatically finish JSONPath expressions.
- Linter and formatter: To ensure consistency throughout your code and to swiftly identify errors, set up a JSON linter and formatter.
4. Build Frameworks for Testing
Incorporate JSONPath testing into your testing frameworks if you’re a part of a larger software project:
- Frameworks for Unit Testing: Use JUnit for Java, pytest for Python, or Jest for JavaScript. Include JSONPath queries in your unit tests to verify JSON outputs.
- Tools for API Testing: You may create tests with JSONPath in programs like Postman to verify the answers to your API queries.
5. Exercise and Examples
To get adept with JSONPath, write queries employing a variety of intricate JSON documents:
- Experimental JSON Data: To practice your JSONPath queries, create or download some sample JSON data.
- Lessons and Assignments: Learn how to handle JSON data by participating in online courses, tutorials, or coding challenges.
6. Sharing and Version Control
If you use JSONPath queries and JSON data samples in a development project, ensure they’re under version control. This procedure facilitates teamwork and keeps track of modifications to your testing scenarios.
7. Continuous Development
Keep up-to-date with the most recent advancements in JSONPath tools, libraries, and syntax. Keeping up with community forums, tool changes, and documentation updates can help maintain a state-of-the-art testing environment.
These procedures will help you create a stable environment ideal for JSONPath testing so that you can effectively manage any activities related to JSON data validation and modification in your projects.
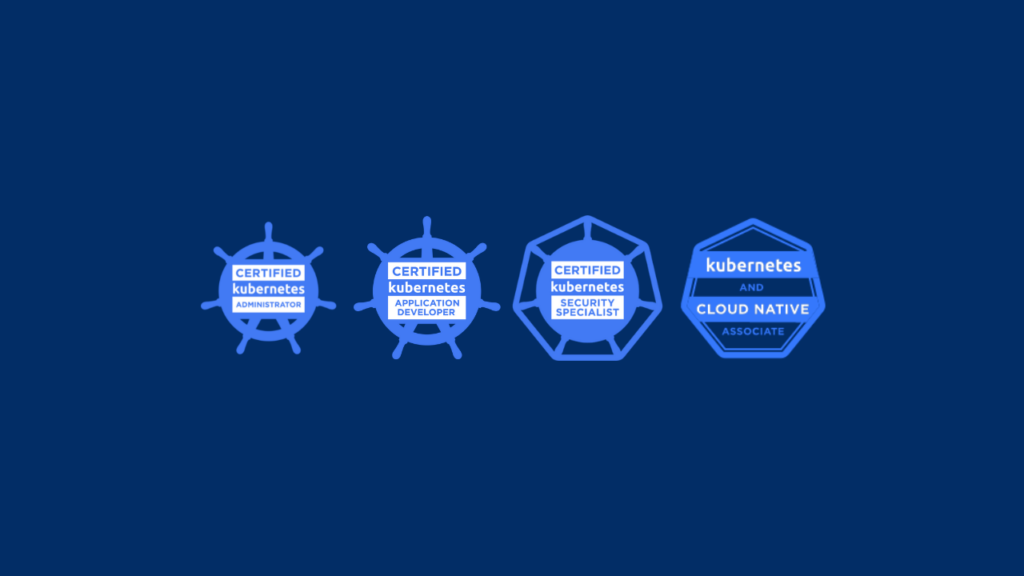
Advanced Methods for Validating Data
Let’s examine a few methods for advanced data validation:
Managing Complicated Data Structures
Advanced data validation requires navigating complex relationships between data points using JSONPath’s capabilities when working with deeply nested or sophisticated JSON structures.
To guarantee the quality and integrity of data across the structure, validators must create exact queries that may extract objects, conditional data, and nested arrays. It frequently entails separating data items according to their relationships or particular requirements inside the data hierarchy using sophisticated operators like recursive descent (..) or filters ([?(@.key > value)]).
JSONPath’s Advanced Operators and Expressions
Several advanced operators and expressions are available in JSONPath, enabling complex JSON data manipulation and querying.
For instance, validation across variable-length arrays or dynamically accessed object attributes can be facilitated by using wildcard expressions (*), slice operators ([start: end]), and script expressions ([(@.length-1)]).
These techniques are beneficial for validations when unique data extraction rules must be applied dynamically based on the data or where data patterns and structures are inconsistent.
- Using JSONPath for Conditional Checks and Nested Data Validation
Conditional checks and nested validations require validators to craft JSONPath queries that assess conditions within the data or span across different layers of the data structure.
It may include validating that certain conditions are met before data is deemed valid, such as checking multiple fields to confirm a dataset’s completeness or integrity.
For instance, JSONPath can verify that all items in an array meet a particular condition or ensure that nested objects adhere to specific organization rules or data quality standards.
A Few Tips For Creating Effective And Efficient JSONPath Queries
When working with JSON data, crafting intelligent and efficient JSONPath queries is crucial to optimizing efficiency and getting precise results. The following insightful advice can help you create more effective JSONPath queries:
- Make It Simple: Formulate the most straightforward query to achieve the intended outcome. If a JSONPath query is overly complex, with more operators or conditions added, debugging will become more challenging, and speed will suffer.
- Employ Precise Path Expressions: Whenever possible, provide more specific paths rather than depending on recursive descent (..), which can search across whole JSON documents or broad wildcards. This method expedites query execution while reducing the volume of data processed.
- Utilize Filters for Specificity: Use filter expressions ([?(@.key==value)]) to fine-tune the data set to precisely what you require. Effective filters for certain conditions can help ensure your queries are as specific as possible and minimize the processing load.
- Avoid Needless Scans: If you are familiar with the structure of your JSON data, use recursive descent only when it is optional. Your searches are substantially slower if you go through every node. Direct lines function best when you are familiar with the organization.
- Validate and Optimize: After creating your query, ensure it is accurate and performs well. Seek methods to simplify expressions or reduce processes to improve the query. Compare various iterations of your query to see which is the most effective.
- Employ Appropriate Indexing in Arrays: When working with arrays, attempt to access elements directly using specified indices ([1], [0:5]), as opposed to sifting through each component. It is significantly faster—especially with big arrays.
AI-powered test orchestration and execution platforms like LambdaTest lets you perform manual and automation testing at scale. Also, it provides a free online JSONPath tester tool. This tool is useful for anyone with JSON data and JSONPath expressions.
It allows you to paste your JSON code and write JSONPath expressions to target specific parts of the data. The tool will then evaluate the expression and show you the extracted information. This can be helpful for debugging your JSONPath queries, learning how to navigate JSON structures, and ultimately working more efficiently with JSON data.
Conclusion
We’ve covered many issues in exploring JSONPath and its uses, from fundamental syntax and functionality to sophisticated data validation strategies and environmental setups for efficient JSONPath testing.
Understanding JSONPath’s fundamental components, the advantages of utilizing JSONPath testers for accurate and rapid data extraction, and sophisticated techniques for managing complicated JSON structures and incorporating JSONPath into development workflows are among the main highlights.